Looking for an internship is a tedious process, requiring loads of patience and determination [1]. Technical interviews usually happen after an initial phone screen, but the progress differs greatly from company to company. For example, Apple has a screening call, two phone interviews, and then an on-site interview whereas Microsoft has a phone interview and then an on-site interview [2]. View Business Insider for more details. Hopefully, since you are looking to prepare for your technical software engineer interview, you have already made some progress in the process and how are looking to preparing for your interviews. Unlike other types of interviews, technical interviews serve to see how you tackle software engineering problems [3]. It’s a chance to prove to your interviewers that you have the skills required for the job through thorough communication and thinking! [3]
If you are interviewing for a specific domain role such as AWS or Machine Learning, then the questions you will be asked will be centered on the specific technology of that domain [4]. In this blog post, we are focusing on a general software engineering intern interview. Before diving into technical interview tips, it’s important to know the basics. Most interviews primarily focus on data structures and algorithms, primarily learned by the beginning of your sophomore year.
Things to Know
To prepare your technical knowledge on data structures and algorithms, these are some concepts and ideas you understand thoroughly.
- Algorithms | You should be able to know how you can approach a problem by finding an algorithm, and how you can improve or change it. Some common algorithms to be familiar with is Dijkstra’s algorithm, binary search, and sorting algorithms, such as quicksort, with a runtime of NlogN.
- Dynamic programming
- Recursion
- Divide and conquer
- Sorting
- Data Structures | You should be very familiar with one programming language such as C++, Java, Python, Go, or C. Be prepared to know how to test your code, come up with edge cases, and utilize your algorithms. Be familiar with object oriented programming concepts.
- Trees
- Linked Lists
- Arrays
- Stacks
- Queues
- Heaps
- Graphs
- Hash Tables / Sets
- Hash Tables / Dictionaries
- Object Oriented Design and Programming | You should be very familiar with one programming language such as C++, Java, Python, Go, or C. Be prepared to know how to test your code, come up with edge cases, and utilize your algorithms. Be familiar with object oriented programming concepts.
- Big-O Notation | It is important to be able to recognize the run-time of any algorithm that you write or any well-known algorithm such as binary search.
- Recursion | Many problems (especially ones with graphs or trees) involve programming in a recursive manner, which can be tricky if not approached properly. It’s important to develop the skills needed to program recursive methods and functions.
- Probability and Combinatorics | You should be familiar with basic discrete math questions. However, these questions are not as frequently used in interview questions at companies other than Google.
List of Topics and Descriptions is from Google Interview Prep Guide
How to Approach a Coding Problem
Though sometimes, you may find yourself using a trial and error approach to solve a coding problem, that is quite the opposite of what these interviewers are looking for. The framework that I encourage you to follow is UPER: Understand, Plan, Execute, Review
Understand – what is the question asking you to do? How is the output desired related to the inputs? Are there any assumptions that you are unsure about? Edge cases? [5]
Plan – Given the desired goal of the problem, how can you achieve it? What is the overall algorithm going to require? What is the main data structure that you need to use? [5]
Learn to write code by hand [6]. An IDE or a keyboard should not be a part of your initial planning. Being able to write code by hand on a whiteboard or paper is crucial because it allows you to focus solely on the algorithm and planning rather than syntax highlighting [6]. In fact, some companies host their technical interviews through Google Docs (such as Google SWE Intern interviews) or through a whiteboard, if it is in person.
Now, you can start typing away
Execute – After your plan, you may execute and write your code – [5]
Depending on the company, this may be pseudocode, an IDE, a whiteboard, etc. Be sure that you can be flexible and execute your “code” in any manner [1].
Review – Check your program with common cases, edge cases, and see if it matches your desired output. If not, then explore where the algorithm may have gone wrong [5].
Communication
Explain how you think to your interviewer. This includes your thought process and all decision making. State and check your assumptions with the interviewer prior to your initial drafting. In fact, they may have open-ended questions on purpose, to see how you engage with the problem and your ability to explain your thought process (Google Interview Prep Guide). This “thinking aloud” allows the interviewer to also see your communication skills as well as your engagement in a productive dialogue [7].
To understand concretely the framework outlined by the UPER method and communication skills, let’s go through this practice coding question.
Example Interview Coding Question
Implement python’s find(), so that it returns the index of the substring in a given string. (Problem from Leetcode)
The interviewer may give you sample cases or they may not. It is totally up to their discretion. In the event that they do not, it is important to ensure that you understood the problem accurately. A sample response could be:
You: Just for clarity, would the word “rabbit” return 2 for the substring “bb”?
Though in this problem, the example cases may be trivial, especially if you are familiar with the find() in Python or strstr() in C or indexOf in Java. However, they may ask you a question where the problem is unfamiliar. In fact, I had a question where I had to represent a number x by the sum of two numbers a, b such that the a and b do not contain any 5’s. Initially, without clarification, I was unsure of what a sample case would be, and whether a and b had to be fixed values, or if any arbitrary two numbers that sum to x yet do not contain any 5’s would suffice.
Another important clarification that should be made is what to return in the case of multiple substrings found or in the case that no substrings are found in the string.
I understand that the function should return the index in the event that the substring is found only once. I am unsure whether this function should return all possible substrings in the case of repetition such as finding “iss” in “Mississippi.” Furthermore, should it return -1 in the case of no found substrings, similar to Python’s find() implementation?
The importance of gaining this clarify prior to thinking about the algorithm is that the interviewer will be able to see that you are considering more than a shallow interpretation of the problem and that you are communicating your assumptions and initial thoughts. I cannot stress enough how much of a significant role that this initial Understand step plays.
def find(haystack, needle):
Though I understand that I need to find every possible substring in the parameter haystack and compare it to the parameter needle, I wonder if knowing that the length of the needle and the length of the substring has to be the equal can help me optimize the problem.
Note that the interviewer may not give you feedback on your every thought or engage in an open dialogue. Do not shy away from this “talking aloud.”
This problem can be thought of as a “window sliding problem” such that we can consider the window pane in a bus [8]. Imagine that the window has a fixed length of n and the pane is fixed at a length of k [8]. Originally, the pane is to the extreme left, and then it is slide over by 1 unit. View the image below where the pane has a length of 3 and the window has a length of 10.
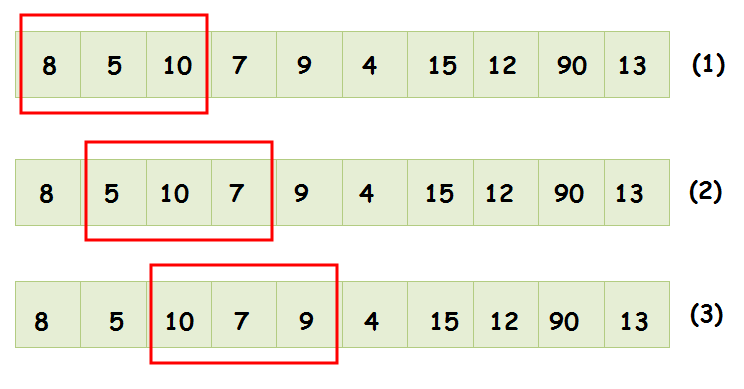
Though understanding a window sliding technique is beside the point of this blog post, it would be helpful to your interviewer if you discussed whether this technique or perhaps another one would be better and discuss why. If you did not know about the “window sliding problem”, do not worry, practice will ensure that you are exposed to different types of problems.
I believe that I can look at the haystack like a ‘window’ and ‘slide’ over by one letter at a time. I think this strategy will give me a runtime of O(N) where N is the number of letters in the haystack.
Utilize the medium between you and the interviewer as a platform to express your thinking through an example test case.
haystack = ‘’rewarding”
needle = “ward”
window 1 : “rewa”
window 2 : “ewar”
window 3 : “ward”
window 4 : “ardi”
window 5 : “rdin”
window 6 : “ding”
Looking at this example, you can explain to the user that this approach would allow you to achieve a linear runtime and look through every possible substring with the same number of characters as the needle.
Below is my implementation in Python.
def find(haystack, needle):
for i in range(0, len(haystack)-len(needle)+1):
if haystack [i:i+len(needle)] == needle:
return i
return -1
Since you may not have an IDE where you may run your code, you should practice verbalizing your test cases. You should state whether you are checking an edge case, and state your desired output before mentally walking through your code.
My test case is going to be: find(“interviewing”, “view”) Now the len(“interviewing”) is equal to 12 and len(“view”) is equal to 4. Thus, I should be looking at the haystack “interview” in 4 character bytes at a time. Initially i is 0, and thus, I am looking at “interviewing”[0:0+4] which is “inte”…
In this problem, edge cases may include where the needle is an empty string, or where the needle is at the very end of the haystack, such as “interaction” and “action.” Telling your interviewer edge cases, and how you believe you should verify that they pass your program shows the interviewer that you are able to thoroughly identify common mistakes made when implementing that particular algorithm.
In the event that any of your test cases fail, it’s important to not panic, and to remember that you are only human. Be honest with your interviewer in how you have trouble identifying the problem and suggest a what part of your code you suspect is leading to the problem. At the end of day, they are looking to hire a thinker not a robot.
Practice
When preparing for coding problems, it’s important that you time yourself [5]. Give yourself an amount of time to attack a problem and be sure you stick with it. Understanding the different types of problems possible will allow you to view a wide variety of genres from which the interviewer can pick from. You may get something similar to your practice or you may not – it does not matter to your interviewer [5].
The goal of practicing isn’t to write flawless code, but rather to attain the knowledge of how you can deal with failures, frustration, and learn how to adapt your thinking [5]. Instead of spending time perfecting your code, you should move to a different problem when you will comfortable with it [5].
By saving several minutes on each problem you will be able to simulate an interview as well. Most interviewers never care about the nitty-gritty details but rather to see if you have the overarching idea and the skills needed to attack and solve the problem with thorough communication.
Technical Questions
Other than extended algorithmic questions, do prepare basic technical questions that indicate your tech knowledge and experience such as some of the questions below. Though there is no necessarily right or wrong answer, it’s important to frame your responses to showcase that you are willing to learn and develop your skills. [10] Some example questions are listed below.
What programming languages have you used?
How do you make sure that your code can handle different kinds of error situations?
What is the difference between re-engineering and reverse engineering?
What is the difference between local and global variables?
Questions all from thebalancecareers.com [10]
When to start Preparing
As a software engineer, practice and preparation will only enhance your problem-solving skills, communication, and analytical skills. Though I recommend one leetcode problem a day to keep your skills sharp, when you begin feeling confident when faced with almost any coding problem is when you know that you are prepared. Typically, this may take between a couple of weeks to a couple of months depending on your skillset and your pace. Best of luck!
Resources
- Cracking the Code Interview, Book
- byte by byte, Website and YouTube
- CS50, YouTube
- Interview Cake, Website
- HackerRank, Website
- LeetCode, Website
Works Cited
[1] “How to Prepare for a Google Internship Interview | by SWE Careers | Medium.” https://medium.com/@swecareers/how-to-prepare-for-a-google-internship-interview-aff644af0d44 (accessed Mar. 30, 2021).
[2] “What It’s Like to Interview at Facebook, Google, Tech Companies.” https://www.businessinsider.com/what-its-like-to-interview-at-facebook-google-and-other-tech-companies-2016-1 (accessed Mar. 30, 2021).
[3] “The Ultimate Guide to Acing Your Technical Interview in 2021 | Learn to Code With Me.” https://learntocodewith.me/posts/technical-interview/#tech-interview-basics (accessed Mar. 11, 2021).
[4] “31 Software Engineering Interview Questions With Answers | Springboard Blog.” https://www.springboard.com/blog/21-software-engineering-interview-questions/ (accessed Mar. 31, 2021).
[5] “How to Practice for Technical Interview Questions | by Michael Chrupcala | codeburst.” https://codeburst.io/how-to-practice-for-technical-interview-questions-b56c2cea02cc (accessed Mar. 11, 2021).
[6] “I just got a developer job at Facebook. Here’s how I prepped for my interviews.” https://www.freecodecamp.org/news/software-engineering-interviews-744380f4f2af/ (accessed Mar. 11, 2021).
[7] “Most Common Technical Interview Questions.” https://www.thebalancecareers.com/top-technical-interview-questions-2061227 (accessed Mar. 11, 2021).
[8] “Window Sliding Technique – GeeksforGeeks.” https://www.geeksforgeeks.org/window-sliding-technique/ (accessed Apr. 05, 2021).
[9] “Maximum Element Sliding Window – Techie Me.” http://techieme.in/maximum-element-sliding-window/ (accessed Apr. 06, 2021).
[10] “Software Engineer Interview Questions.” https://www.thebalancecareers.com/software-engineer-interview-questions-2063875 (accessed Mar. 11, 2021).
Featured Image from https://sites.temple.edu/careercentercontent/