Last updated on August 3, 2021
According to the 2020 Stack Overflow Developer Survey, JavaScript is the second most wanted programming language after Python [1]. JavaScript is crucial in the creation of dynamic interactive web applications. The programming language allows developers to code simple tasks– like making drop-down menus– as well as more advanced features, like accessing a user’s browser history. In fact, JavaScript is used in some capacity by over 94% of websites [2]. If you are an experienced coder seeking to try out JavaScript for the first time, you are in the right place.
In this post, we will go over some basic tips on JavaScript for those with prior coding experience. The goal of this post is to provide a basic history of JavaScript, an overview of its elementary syntax and features, and resources for additional study.
Context
JavaScript was born in 1995, when the company Netscape Communications wanted to develop a scripting language that would complement Java and help implement dynamic web functionality, interactive applications, and server-side tasks. The company Ecma International helped Netscape standardize the language, and today, Ecma International and the Mozilla Foundation are responsible for the development and upkeep of this language [3].
Modern JavaScript is responsible for many important features of websites, from autocompletion to video players. Today, many JavaScript programmers use frameworks like NodeJS, AngularJS, or ReactJS, all of which are offshoots of the original language [4]. Now, with an understanding of what this language is and why it exists, we can jump into its code.
Commenting and Printing
Two crucial skills in any programmer’s toolkit are printing and commenting. In JavaScript, we print using the function console.log(). The expression or literal we are printing goes in the parentheses. String literals must be enclosed in single or double-quotes.
JavaScript also allows developers to write single- or multi-line comments. Single-line comments begin with a double forward-slash (//) and end automatically on the new line. Multi-line comments can be any number of lines and must begin with /* and end with */. Examples are shown below.
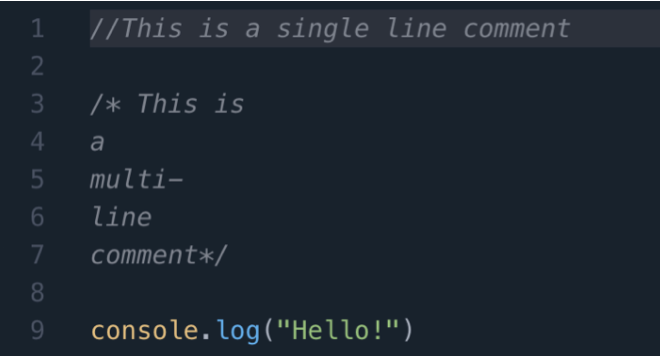
Variables
JavaScriptcontains many common primitive types, like strings, booleans, ints, floats, and others. Variables can also be null, meaning that they have been assigned to have no value, or undefined, meaning that they have not yet been assigned a value. JavaScript variables can also be instances of a user-defined class.
Variable names cannot start with numbers, and variable names also cannot be the same as any JavaScript keyword (for example, def, break, or continue).
You can define a variable in JavaScript using one of three keywords: var, let, and const. Originally, var was the only keyword for variable declaration [5]. JavaScript later introduced the const and let keywords because var has a quirk that is confusing to developers: a var of the same name can be redeclared within its scope. As a result, programmers would sometimes accidentally redeclare a variable, not realizing there was already another variable of the same name in their code, causing unexpected behavior. Developers kept the var keyword so that legacy code would still run, so you will still see the var keyword used, but should use let and const whenever possible.
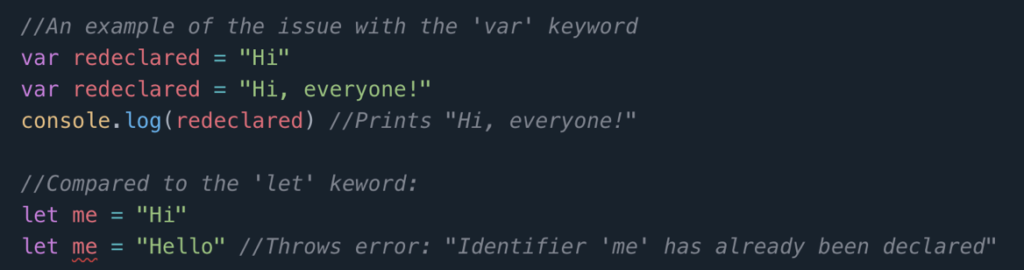
Let us walk through an example by defining two variables, one declared using let, the other declared using const. Both variables will be scoped by block, but the variable declared using the let keyword can be updated, and the one declared using the const cannot be updated– its value is fixed. Neither variable can be redeclared as var can. Confusingly, while you cannot reassign a const object, you can update its properties individually [6].
Conditionals
Most programmers are familiar with the idea of the conditional statements JavaScript includes, such as if/else statements, switch statements, and catch/throw statements. For more information on syntax and some examples, see the MDN Web Doc’s section on Conditionals.
What differentiates JavaScript is how it decides whether a conditional statement is true or false. Javascript distinguishes between “truthy” and “falsy” values for many different types. “Falsy” values include the number 0, the Boolean false, any undefined or null value, NaN (not a number), and the empty string (“”). Anything that is not a falsy value is a truthy value– so using an instance of an object that is not null or undefined as a conditional statement always evaluates to true [7].
In addition to “truthy” and “falsy” variables, JavaScript also supports the more traditional use of operators like the logical AND (denoted &&), the inequality operators (denoted !=), and the logical OR (denoted ||). Each of these operators can be used to define a conditional for an if/else statement or other conditional statement.
Also important to note is the difference between equality and strict equality. The equality operator (==) and the inequality operator (!=) attempt to make the data types the same before checking for equality, while the identity operator (===) requires the data types to be the same to evaluate to true. If you’re not careful, these operators can lead to unexpected behavior in your program, so it’s important to be selective [7].
Shown below is an example of a simple if/else statement using truthy and falsy values.
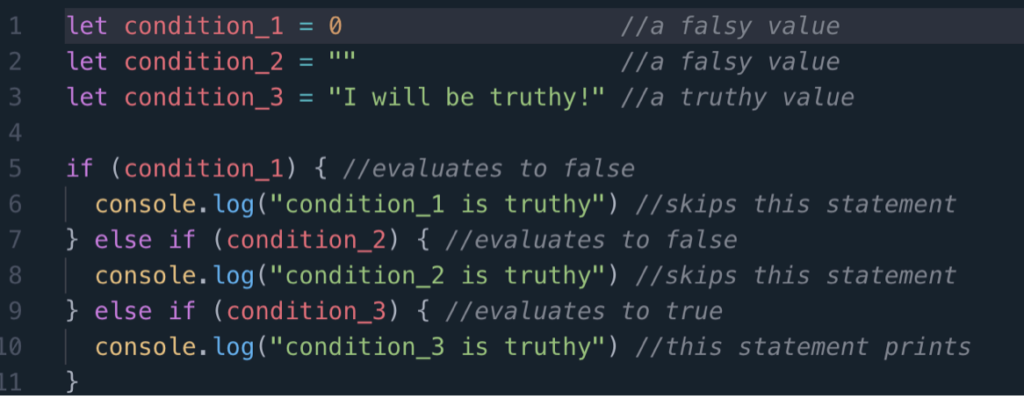
Loops
Similar to Java, for-loops in JS require an initial expression, a condition expression, and an increment expression. It runs the loop body each time it increments the initial expression until the condition expression is met. While-loops require only a condition expression and will run infinitely if the condition expression is never met.
Like Python’s for…in loop, JavaScript allows users to iterate through iterable types like Arrays directly with its for…of loop. An important distinction to make is that JavaScript does also contain a for…in loop; however, that loop iterates through enumerable properties of an object, not elements in a list or array, and will return an error if used to iterate through a collection like an Array [8].
JavaScript also allows the programmer to direct loops using the keywords break and continue. The break keyword tells the user to exit the loop immediately, and the continue keyword tells the user to skip to the next iteration of the loop, unless the loop condition is false, in which case the computer will exit the loop.
Programmers can use labels in their program to differentiate between nested loops, allowing the user to specify with these keywords which specific loop they would like to break or continue [8].
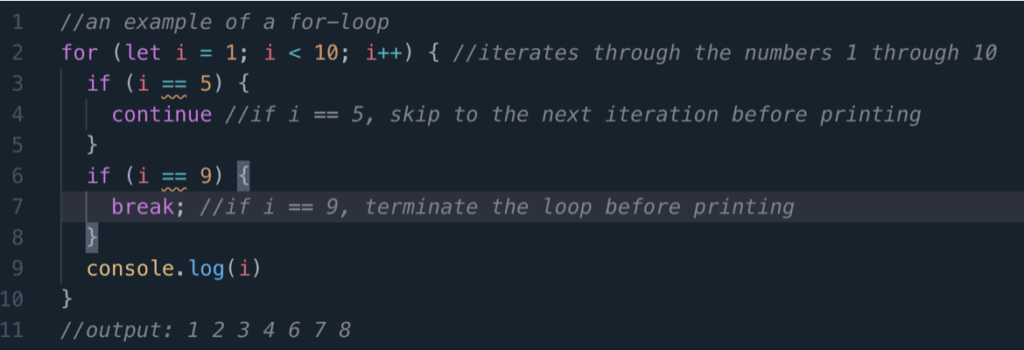
Functions
Functions in JavaScript follow a similar format as those in Python. Their headers begin with the keyword def, the function’s name, and its parameters. The function body is enclosed by brackets. A function has access to all variables and functions defined within its scope [5].
Like in Python (but unlike Java), the programmer does not need to specify the types of the parameters. The programmer also does not specify whether the function has a return type in the function header.

Function Expressions and Arrow Function Expressions
Similar to Swift, JavaScript also allows the programmer to declare a function without using a traditional function header. These are called in-line functions. To declare an in-line function, the programmer uses the function keyword. An in-line function may not have a name, in which case it is called an anonymous function [9].
JavaScript also allows for the use of arrow functions, which are always anonymous (unnamed). They are often used as arguments for other functions. Arrow functions are complex, and we will not cover them in-depth in this post, but you can read more about them here. Below are a few examples of in-line and anonymous functions.
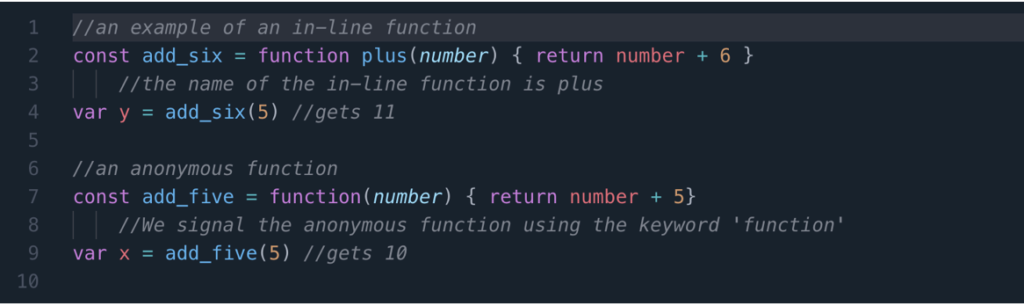
Additional Resources
As previously mentioned, the goal of this blog post is to provide some background and elementary tips on coding in JavaScript. To continue your learning, consider working through some of the following resources.
- Codecademy’s Javascript class, a free online course that teaches the basics of JavaScript. While aimed at beginner programmers, an experienced coder can zip through this course while picking up important tips on the language. The advantage of a course– whether this one or another like those offered by FreeCodeCamp or Udemy– is that in addition to learning to read JavaScript, you have the opportunity to try graded coded exercises yourself as you go along, helping cement these Javascript skills as you practice them.
- The MDN Web Docs’ Javascript Guide. The documentation here is clear, concise, and well-organized, and can help clarify the finer points of JavaScript’s syntax and structure to the elementary user.
- The book JavaScript and JQuery: Interactive Front-End Web Development. For those interested in using JavaScript for web development and design purposes, this book is handy and user-friendly, with lots of graphics and colors, while also being incredibly informative, full of information on how to best harness JavaScript to create beautiful, functional web applications
- Maintainable JavaScript, a book that helps Javascript programmers write code that is not only functional, but readable, a skill that is crucial when working on big projects or at large companies. This is a great read for anyone looking to be in the field of Javascript long-term.
With the above background and these additional resources in tow, you are well on your way to becoming a proficient Javascript developer. If you enjoyed this blog post and began your JavaScript journey I’d love to hear about your experiences and tips. Contact me at ceo2129@barnard.edu. Good luck!
Sources
[4] “JavaScript | MDN.” https://developer.mozilla.org/en-US/docs/Web/JavaScript.